I was working on a website which featured a video on the home page. The home page video loaded quickly but I wanted to ensure if there was a connection delay the user would not be presented with a blank screen.
I added a function to WordPress to substitute a placeholder static image with the video once the video was available. I coded a one second delay before calling the function.
If the video wasn’t available the user would be presented with a screen filled with a static image and allowed to continue the navigation.
This function will examine the home page and look for an alt tag ‘video-placeholder’ and once the page is loaded it will replace the placeholder with the video if available.
Home Page Video Function
function replace_image_with_video_homepage() {
if (is_front_page() || is_home()) {
?>
<script>
document.addEventListener("DOMContentLoaded", function () {
var placeholder = document.querySelector('img[alt="video-placeholder"]');
if (placeholder) {
console.log("Placeholder found, waiting before replacing with video...");
// Add a delay of 1000ms (1 second) before replacing the image
setTimeout(function () {
var video = document.createElement('video');
video.setAttribute('class', 'wp-block-cover__video-background intrinsic-ignore');
video.setAttribute('autoplay', '');
video.setAttribute('muted', '');
video.muted = true; // Ensure it's muted
video.setAttribute('loop', '');
video.setAttribute('playsinline', '');
video.setAttribute('data-object-fit', 'cover');
video.setAttribute('src', 'your url for the video');
video.addEventListener('loadeddata', function () {
console.log("Video loaded, replacing placeholder.");
placeholder.replaceWith(video);
// Force autoplay attempt
video.play().then(() => {
console.log("Video autoplay successful.");
}).catch(error => {
console.log("Autoplay failed:", error);
});
});
video.load();
}, 1000); // Delay of 1 second (1000ms)
} else {
console.log("Placeholder image not found.");
}
});
</script>
<?php
}
}
add_action('wp_footer', 'replace_image_with_video_homepage');
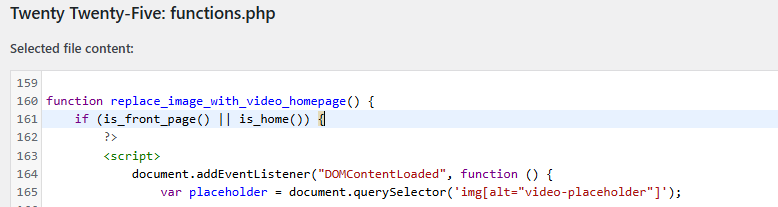
I added the code to the functions.php in WordPress. If you update the theme you will need to add the code as this will be overwritten. You can also use ‘Code Snippets‘ plugin and add this as a php function which will be retained as you run updates.
On the console log (F12 then select console on chrome) you can see the builtin messages in the function to troubleshoot if necessary. Screen shot below
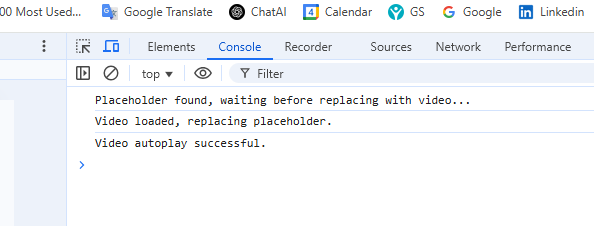
Check out my other wordpress posts
There are a number of ways to deal with preloading in wordpress.