Manage your site downloads ; this plugin will load all forms into a single folder and allow easy display in alphabetical order on any page or post on your site.
From the admin menu you can load the file and give it a description which can be edited if required or removed if no longer required.
To list all the documents on your site you can use the sort code
which will shows the forms on your site.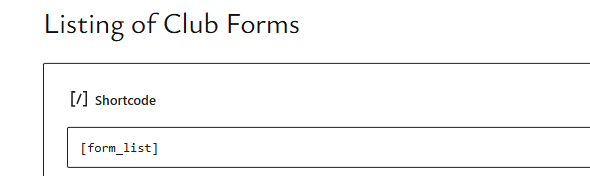
Load this file into your plugin folder and activate it using WordPress admin.
Manage your Site Downloads – Plugin Code
<?php
/**
* Plugin Name: WP Forms Manager
* Description: Allows admin users to upload forms, add descriptions, and display them via a shortcode.
* Version: 1.0
* Author: Anything Access
* Author URI: https://anythingaccess.com
*/
// Create forms directory on plugin activation
function wpfm_create_forms_directory() {
$upload_dir = WP_CONTENT_DIR . '/forms';
if (!file_exists($upload_dir)) {
mkdir($upload_dir, 0755, true);
}
}
register_activation_hook(__FILE__, 'wpfm_create_forms_directory');
// Create database table for storing form details
function wpfm_create_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'forms_manager';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id MEDIUMINT NOT NULL AUTO_INCREMENT,
file_name VARCHAR(255) NOT NULL,
description TEXT NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
register_activation_hook(__FILE__, 'wpfm_create_table');
// Add admin menu
function wpfm_admin_menu() {
add_menu_page('Forms Manager', 'Forms Manager', 'manage_options', 'wpfm', 'wpfm_admin_page');
}
add_action('admin_menu', 'wpfm_admin_menu');
// Admin page HTML
function wpfm_admin_page() {
global $wpdb;
$table_name = $wpdb->prefix . 'forms_manager';
if (isset($_POST['upload_form'])) {
if (!empty($_FILES['form_file']['name'])) {
$upload_dir = WP_CONTENT_DIR . '/forms/';
$file_name = basename($_FILES['form_file']['name']);
$file_path = $upload_dir . $file_name;
if (move_uploaded_file($_FILES['form_file']['tmp_name'], $file_path)) {
$description = sanitize_text_field($_POST['description']);
$wpdb->insert($table_name, ['file_name' => $file_name, 'description' => $description]);
echo '<div class="updated"><p>Form uploaded successfully!</p></div>';
} else {
echo '<div class="error"><p>File upload failed.</p></div>';
}
}
}
if (isset($_GET['delete'])) {
$id = intval($_GET['delete']);
$file = $wpdb->get_var($wpdb->prepare("SELECT file_name FROM $table_name WHERE id = %d", $id));
@unlink(WP_CONTENT_DIR . '/forms/' . $file);
$wpdb->delete($table_name, ['id' => $id]);
echo '<div class="updated"><p>Form deleted successfully!</p></div>';
}
if (isset($_POST['edit_form'])) {
$id = intval($_POST['form_id']);
$description = sanitize_text_field($_POST['description']);
$wpdb->update($table_name, ['description' => $description], ['id' => $id]);
echo '<div class="updated"><p>Description updated successfully!</p></div>';
}
$forms = $wpdb->get_results("SELECT * FROM $table_name ORDER BY file_name ASC");
?>
<div class="wrap">
<h2>Forms Manager</h2>
<form method="post" enctype="multipart/form-data">
<table>
<tr><td>Description:</td><td><input type="text" name="description" style="width: 400px;" required></td></tr>
<tr><td>Upload File:</td><td><input type="file" name="form_file" required></td></tr>
</table>
<input type="submit" name="upload_form" value="Upload" class="button button-primary">
</form>
<hr>
<h3>Uploaded Forms</h3>
<table class="widefat">
<thead><tr><th>Description</th><th>File</th><th>Actions</th></tr></thead>
<tbody>
<?php foreach ($forms as $form) { ?>
<tr>
<td>
<form method="post" style="display:inline;">
<input type="hidden" name="form_id" value="<?php echo $form->id; ?>">
<input type="text" name="description" value="<?php echo esc_html($form->description); ?>" style="width: 400px;">
<input type="submit" name="edit_form" value="Save" class="button button-secondary">
</form>
</td>
<td><a href="<?php echo content_url('/forms/' . esc_attr($form->file_name)); ?>" target="_blank"><?php echo esc_html($form->file_name); ?></a></td>
<td><a href="?page=wpfm&delete=<?php echo $form->id; ?>" onclick="return confirm('Delete this form?');">Delete</a></td>
</tr>
<?php } ?>
</tbody>
</table>
</div>
<?php
}
// Shortcode to display forms list
function wpfm_shortcode() {
global $wpdb;
$table_name = $wpdb->prefix . 'forms_manager';
$forms = $wpdb->get_results("SELECT * FROM $table_name ORDER BY file_name ASC");
if (empty($forms)) return '<p>No forms available.</p>';
$output = '<ul>';
foreach ($forms as $form) {
$url = content_url('/forms/' . esc_attr($form->file_name));
$output .= '<li><a href="' . esc_url($url) . '" target="_blank">' . esc_html($form->description) . '</a></li>';
}
$output .= '</ul>';
return $output;
}
add_shortcode('form_list', 'wpfm_shortcode');
You can view our other wordpress sample here