I wanted a image slider for WordPress that scanned a folder and presented the images to a maximum size with the user option to view and view the image in its original format. If a image was smaller than the container the object wouldn’t distort.
The user can automatically scan a given folder and log all the images in a table. This was written as a plugin with a shortcode to show the slider whever you wished on your website.
I used ChatGPT to code sections of this plugin
This was implemented for Tramore Golf Club on this page.
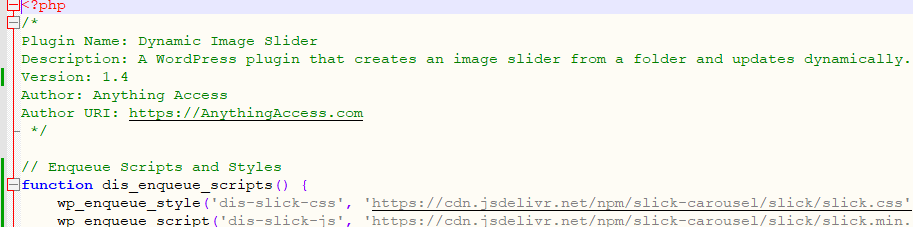
Using this option you have an admin screen which will allow you to scan the selected folder – hard coded to slider-images in the upload directory. And any new images are added to the table.
You have the option to add a description which is shown below the image preview.
This is show the plugin looks in action.
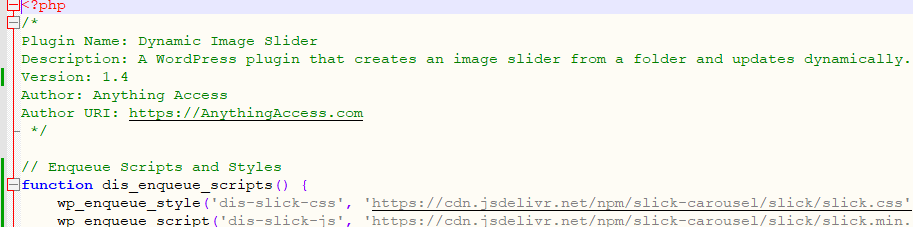
Image Slider Plugin for Wordpress
<?php
/*
Plugin Name: Dynamic Image Slider
Description: A WordPress plugin that creates an image slider from a folder and updates dynamically.
Version: 1.5
Author: Anything Access
Author URI: https://AnythingAccess.com
*/
// Enqueue Scripts and Styles
function dis_enqueue_scripts() {
wp_enqueue_style('dis-slick-css', 'https://cdn.jsdelivr.net/npm/slick-carousel/slick/slick.css');
wp_enqueue_script('dis-slick-js', 'https://cdn.jsdelivr.net/npm/slick-carousel/slick/slick.min.js', array('jquery'), null, true);
wp_enqueue_script('dis-slider-script', plugins_url('slider.js', __FILE__), array('jquery', 'dis-slick-js'), null, true);
wp_localize_script('dis-slider-script', 'dis_ajax', array('ajax_url' => admin_url('admin-ajax.php')));
}
add_action('wp_enqueue_scripts', 'dis_enqueue_scripts');
// Shortcode for Image Slider
function dis_image_slider_shortcode() {
ob_start();
?>
<div id="image-slider-container">
<div class="image-slider">
<?php echo dis_get_stored_images(); ?>
</div>
<button class="slider-prev">❮</button>
<button class="slider-next">❯</button>
</div>
<?php
return ob_get_clean();
}
add_shortcode('image_slider', 'dis_image_slider_shortcode');
// Store Image Paths in Database (Only Add New Images)
function dis_store_images() {
global $wpdb;
$table_name = $wpdb->prefix . 'dis_images';
error_log(wp_upload_dir()['basedir'] . '/slider-images');
$folder = wp_upload_dir()['basedir'] . '/slider-images';
$url_base = wp_upload_dir()['baseurl'] . '/slider-images';
$images = glob($folder . '/*.{jpg,jpeg,png,gif}', GLOB_BRACE);
error_log(print_r($images, true));
foreach ($images as $image) {
$img_url = $url_base . '/' . basename($image);
// Check if image already exists
$exists = $wpdb->get_var($wpdb->prepare("SELECT COUNT(*) FROM $table_name WHERE image_url = %s", $img_url));
if (!$exists) {
$wpdb->insert($table_name, array('image_url' => $img_url, 'description' => ''));
}
}
}
// Retrieve Stored Images
function dis_get_stored_images() {
global $wpdb;
$table_name = $wpdb->prefix . 'dis_images';
$images = $wpdb->get_results("SELECT image_url, description FROM $table_name");
$html = '';
foreach ($images as $image) {
$html .= "<div class='slider-item'><a href='{$image->image_url}' target='_blank'><img src='{$image->image_url}' style='max-width:768px;max-height:432px;'/></a><p class='image-description'>{$image->description}</p></div>";
}
return $html;
}
// Create Table on Plugin Activation
function dis_create_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'dis_images';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id mediumint(9) NOT NULL AUTO_INCREMENT,
image_url text NOT NULL,
description text NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once ABSPATH . 'wp-admin/includes/upgrade.php';
dbDelta($sql);
}
register_activation_hook(__FILE__, 'dis_create_table');
// Admin Panel for Editing Image Descriptions and Refresh Button
function dis_admin_edit_images() {
global $wpdb;
$table_name = $wpdb->prefix . 'dis_images';
$images = $wpdb->get_results("SELECT * FROM $table_name");
echo '<div class="wrap"><h2>Edit Image Descriptions</h2>';
echo '<form method="post">';
foreach ($images as $image) {
echo "<div><img src='{$image->image_url}' style='width:100px;height:auto;'><br/>";
echo "<input type='text' name='desc[{$image->id}]' value='{$image->description}' /><br/></div>";
}
echo '<input type="submit" name="update_descriptions" value="Save Changes">';
echo '</form>';
echo '<h3>Refresh Image List</h3>';
echo '<button id="dis-refresh-images">Refresh Images</button>';
echo '</div>';
if (isset($_POST['update_descriptions'])) {
foreach ($_POST['desc'] as $id => $desc) {
$wpdb->update($table_name, array('description' => sanitize_text_field($desc)), array('id' => $id));
}
echo '<p>Descriptions updated.</p>';
}
}
// AJAX Refresh Button Handler
function dis_refresh_images_ajax() {
dis_store_images();
echo 'Image list updated.';
wp_die();
}
add_action('wp_ajax_dis_refresh_images', 'dis_refresh_images_ajax');
function dis_admin_menu() {
add_menu_page('Image Slider', 'Image Slider', 'manage_options', 'dis-image-slider', 'dis_admin_edit_images');
}
add_action('admin_menu', 'dis_admin_menu');
// JavaScript for Refresh Button
function dis_add_script_file() {
$script = "
jQuery(document).ready(function($) {
$('#dis-refresh-images').click(function() {
$.post(dis_ajax.ajax_url, { action: 'dis_refresh_images' }, function(response) {
alert(response);
location.reload();
});
});
});
";
file_put_contents(plugin_dir_path(__FILE__) . 'slider.js', $script);
}
dis_add_script_file();
For more Wordpress plugins click here